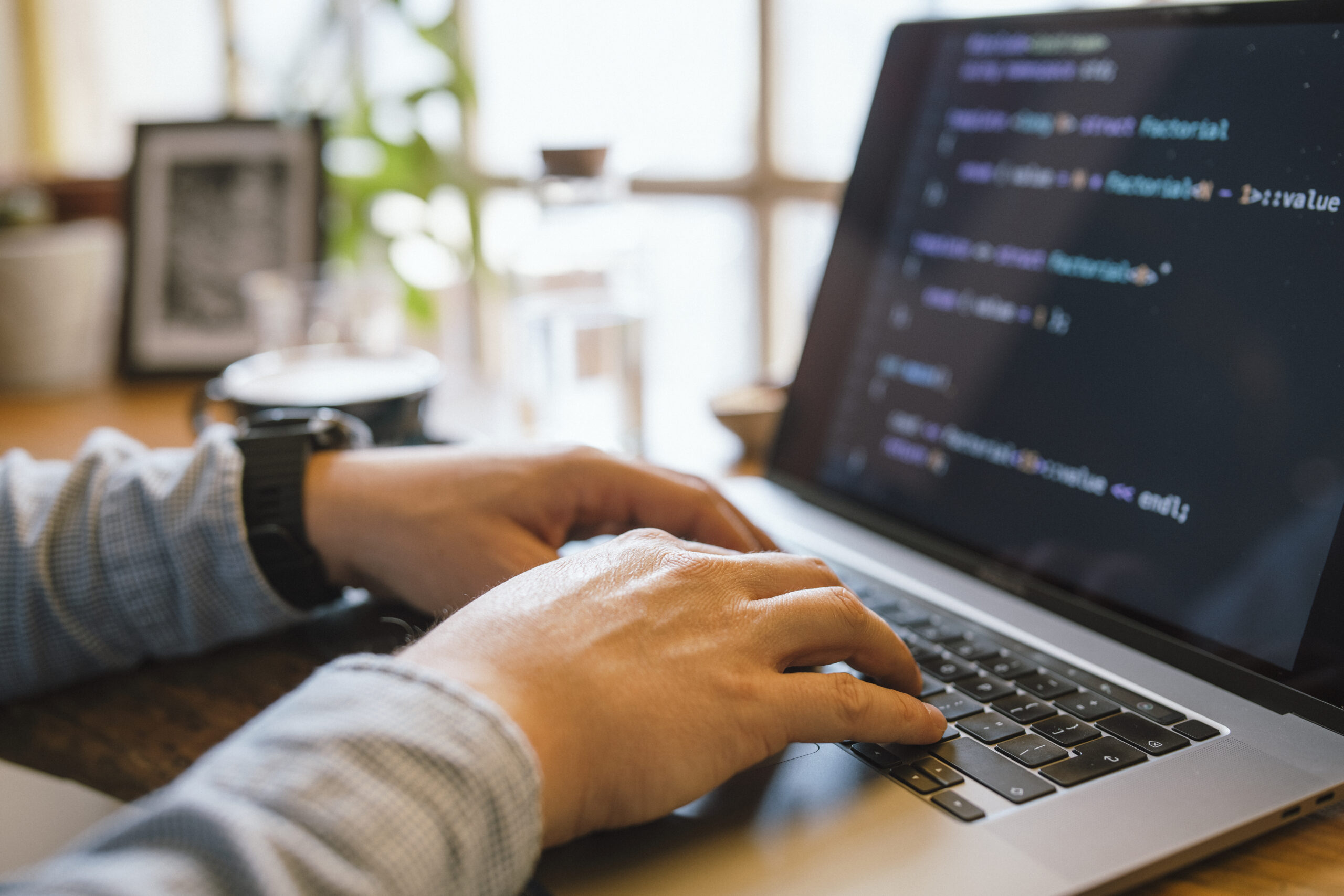
Debugging is Among the most necessary — but generally missed — skills inside a developer’s toolkit. It isn't pretty much correcting damaged code; it’s about understanding how and why things go Incorrect, and Understanding to Assume methodically to unravel challenges competently. Whether you are a starter or perhaps a seasoned developer, sharpening your debugging abilities can save hours of frustration and dramatically improve your efficiency. Allow me to share many techniques to aid developers amount up their debugging activity by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest techniques developers can elevate their debugging competencies is by mastering the tools they use every day. Though producing code is a single A part of development, understanding how to connect with it efficiently throughout execution is Similarly critical. Contemporary growth environments arrive Geared up with strong debugging capabilities — but quite a few developers only scratch the surface of what these applications can do.
Take, one example is, an Integrated Enhancement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools enable you to set breakpoints, inspect the worth of variables at runtime, step by code line by line, and in many cases modify code within the fly. When made use of appropriately, they Permit you to observe accurately how your code behaves all through execution, which can be a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end builders. They allow you to inspect the DOM, keep an eye on community requests, check out authentic-time overall performance metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into workable duties.
For backend or procedure-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management around jogging procedures and memory management. Understanding these instruments may have a steeper Studying curve but pays off when debugging functionality problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into comfortable with version Handle programs like Git to be familiar with code historical past, come across the precise instant bugs were being released, and isolate problematic changes.
In the long run, mastering your instruments usually means likely further than default settings and shortcuts — it’s about developing an intimate familiarity with your progress ecosystem to make sure that when issues arise, you’re not lost in the dark. The higher you understand your equipment, the more time you are able to invest solving the actual difficulty as opposed to fumbling by means of the process.
Reproduce the Problem
One of the most critical — and infrequently ignored — actions in effective debugging is reproducing the condition. Right before leaping to the code or generating guesses, builders want to create a consistent ecosystem or state of affairs the place the bug reliably appears. Without having reproducibility, fixing a bug results in being a video game of likelihood, frequently bringing about wasted time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question concerns like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The greater depth you have, the much easier it gets to be to isolate the precise situations less than which the bug happens.
After you’ve gathered adequate information, try and recreate the problem in your neighborhood environment. This may suggest inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, think about producing automated exams that replicate the sting cases or condition transitions associated. These assessments don't just aid expose the situation but also avoid regressions Down the road.
Occasionally, The problem may be ecosystem-particular — it would transpire only on certain working programs, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a mindset. It demands persistence, observation, and also a methodical solution. But after you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging equipment far more proficiently, take a look at probable fixes properly, and converse additional Evidently with all your workforce or buyers. It turns an summary criticism right into a concrete problem — and that’s the place builders thrive.
Study and Comprehend the Error Messages
Mistake messages are often the most beneficial clues a developer has when some thing goes Improper. Instead of seeing them as frustrating interruptions, builders really should understand to deal with error messages as direct communications from the procedure. They generally let you know precisely what happened, where by it happened, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the concept cautiously As well as in full. Quite a few developers, specially when underneath time stress, glance at the 1st line and quickly start off creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste error messages into search engines — read through and comprehend them to start with.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These questions can tutorial your investigation and point you toward the liable code.
It’s also beneficial to be familiar with the terminology on the programming language or framework you’re applying. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Understanding to acknowledge these can drastically quicken your debugging course of action.
Some errors are imprecise or generic, As well as in Individuals scenarios, it’s crucial to examine the context through which the mistake occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede much larger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Mastering to interpret them appropriately turns chaos into clarity, supporting you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is Probably the most potent instruments inside of a developer’s debugging toolkit. When made use of effectively, it provides real-time insights into how an software behaves, helping you understand what’s happening beneath the hood with no need to pause execution or phase throughout the code line by line.
An excellent logging method begins with understanding what to log and at what level. Typical logging stages contain DEBUG, Information, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information and facts all through development, Facts for typical gatherings (like profitable start off-ups), WARN for potential challenges that don’t split the appliance, ERROR for actual complications, and Lethal once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. A lot of logging can obscure essential messages and decelerate your technique. Concentrate on key gatherings, condition changes, enter/output values, and significant choice details within your code.
Format your log messages Plainly and constantly. Include context, for instance timestamps, request IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-assumed-out logging method, you may reduce the time it will require to identify problems, achieve further visibility into your programs, and Enhance the In general maintainability and reliability of your respective code.
Think Like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently identify and repair bugs, builders will have to method the method just like a detective fixing a secret. This mentality helps break down sophisticated challenges into workable parts and adhere to clues logically to uncover the basis result in.
Start off by collecting proof. Consider the signs or symptoms of the trouble: error messages, incorrect output, or efficiency troubles. The same as a detective surveys a criminal offense scene, accumulate just as much applicable information as you are able to devoid of leaping to conclusions. Use logs, take a look at conditions, and person stories to piece jointly a transparent photo of what’s occurring.
Upcoming, type hypotheses. Inquire your self: What might be creating this behavior? Have any changes recently been produced to the codebase? Has this problem occurred prior to under similar circumstances? The intention will be to slim down prospects and identify opportunity culprits.
Then, take a look at your theories systematically. Seek to recreate the situation within a controlled ecosystem. In case you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, question your code queries and Enable the final results lead you nearer to the truth.
Pay back near attention to smaller specifics. Bugs usually disguise while in the least predicted locations—similar to a missing semicolon, an off-by-just one error, or maybe a race problem. Be thorough and affected individual, resisting the urge to patch the issue devoid of totally being familiar with it. Short-term fixes may perhaps conceal the true problem, only for it to resurface later.
And finally, keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future troubles and assistance Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in intricate units.
Create Exams
Producing checks is among get more info the most effective methods to increase your debugging techniques and overall improvement performance. Checks don't just help catch bugs early but additionally serve as a safety Internet that provides you self confidence when building variations for your codebase. A effectively-examined application is simpler to debug since it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device tests, which concentrate on person functions or modules. These small, isolated tests can quickly expose irrespective of whether a selected bit of logic is Performing as predicted. Any time a exam fails, you straight away know wherever to glance, drastically minimizing time invested debugging. Device checks are Specially valuable for catching regression bugs—difficulties that reappear immediately after Formerly becoming fixed.
Future, combine integration exams and end-to-close assessments into your workflow. These aid make sure that various parts of your software perform with each other effortlessly. They’re notably beneficial for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you'll need to be familiar with its inputs, anticipated outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug could be a robust first step. After the exam fails regularly, you may focus on repairing the bug and enjoy your test move when The problem is fixed. This method makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch far more bugs, speedier and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer soon after Resolution. But One of the more underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen annoyance, and infrequently see The difficulty from the new point of view.
When you are way too near to the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain turns into significantly less effective at dilemma-fixing. A short wander, a espresso split, and even switching to a distinct activity for 10–quarter-hour can refresh your concentration. Quite a few developers report discovering the root of a dilemma after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Specifically throughout longer debugging classes. Sitting before a display screen, mentally stuck, is not just unproductive but also draining. Stepping absent permits you to return with renewed energy in addition to a clearer frame of mind. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment split. Use that point to move all over, stretch, or do a thing unrelated to code. It might sense counterintuitive, Specifically under restricted deadlines, but it in fact leads to more rapidly and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak spot—it’s a smart method. It presents your brain Room to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you face is a lot more than just A brief setback—It is really an opportunity to expand being a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you some thing useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by inquiring yourself a couple of important queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code assessments, or logging? The responses often expose blind places inside your workflow or comprehending and enable you to Construct more powerful coding behavior shifting forward.
Documenting bugs may also be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see designs—recurring concerns or frequent errors—that you can proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically potent. Whether it’s via a Slack concept, a short generate-up, or a quick expertise-sharing session, assisting others stay away from the identical issue boosts staff efficiency and cultivates a much better Finding out tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary elements of your enhancement journey. All things considered, several of the best builders are not the ones who write best code, but those who continually learn from their problems.
In the end, Every single bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll occur away a smarter, far more able developer due to it.
Summary
Improving upon your debugging expertise usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be greater at That which you do.